コード
GoogleDrive上にGASのファイルを作成して、以下のコードを記述する。
// 変換したいファイルが保存されているフォルダID
const docId = 'XXXXXXX';
// 変換したファイルを保存するフォルダID
const folderId = 'XXXXXXX';
function toPDFs() {
let doc_Folder = DriveApp.getFolderById(docId);
let pdf_Folder = DriveApp.getFolderById(folderId);
convertFolderToPDF(doc_Folder, pdf_Folder);
}
function convertFolderToPDF(sourceFolder, destinationFolder) {
let files = sourceFolder.getFiles();
while (files.hasNext()) {
var buff = files.next();
try {
var doc;
var pdf_name = buff.getName() + '.pdf';
// 変換先のファイルが既に存在する場合は変換をスキップ
if (destinationFolder.getFilesByName(pdf_name).hasNext()) {
console.log('Skipped: ' + buff.getName());
continue;
}
if (buff.getMimeType() === 'application/vnd.google-apps.document') {
// Googleドキュメント形式のファイルを開く
doc = DocumentApp.openById(buff.getId());
} else if (buff.getMimeType() === 'application/vnd.openxmlformats-officedocument.wordprocessingml.document') {
// Word形式のファイルを一時的にGoogleドキュメント形式に変換して開く
var tempDocFile = DriveApp.createFile(buff.getBlob());
var tempDocId = Drive.Files.insert(
{
title: tempDocFile.getName(),
mimeType: 'application/vnd.google-apps.document',
},
tempDocFile.getBlob(),
{
convert: true,
}
).id;
doc = DocumentApp.openById(tempDocId);
tempDocFile.setTrashed(true); // 一時ファイルをゴミ箱に移動する
} else {
console.log('Unsupported file format: ' + buff.getName());
continue;
}
// PDFに変換して保存
destinationFolder.createFile(doc.getAs('application/pdf')).setName(pdf_name);
console.log('OK: ' + buff.getName());
} catch (e) {
console.log(buff.getName());
console.log(e);
}
}
let subFolders = sourceFolder.getFolders();
while (subFolders.hasNext()) {
let subFolder = subFolders.next();
let newDestinationFolder = getOrCreateSubFolder(destinationFolder, subFolder.getName());
convertFolderToPDF(subFolder, newDestinationFolder);
}
}
function convertToPDF(file) {
let doc;
let mimeType = file.getMimeType();
if (mimeType === "application/vnd.google-apps.document") {
doc = DocumentApp.openById(file.getId());
} else if (mimeType === "application/msword") {
let docFile = DriveApp.createFile(file.getBlob());
doc = DocumentApp.openById(docFile.getId());
docFile.setTrashed(true);
} else {
console.log('Invalid file format: ' + file.getName());
return null;
}
return doc.getAs("application/pdf");
}
function getOrCreateSubFolder(parentFolder, subFolderName) {
let subFolders = parentFolder.getFoldersByName(subFolderName);
if (subFolders.hasNext()) {
return subFolders.next();
} else {
return parentFolder.createFolder(subFolderName);
}
}
設定
以下のように、サービス>Drive APIを追加する。
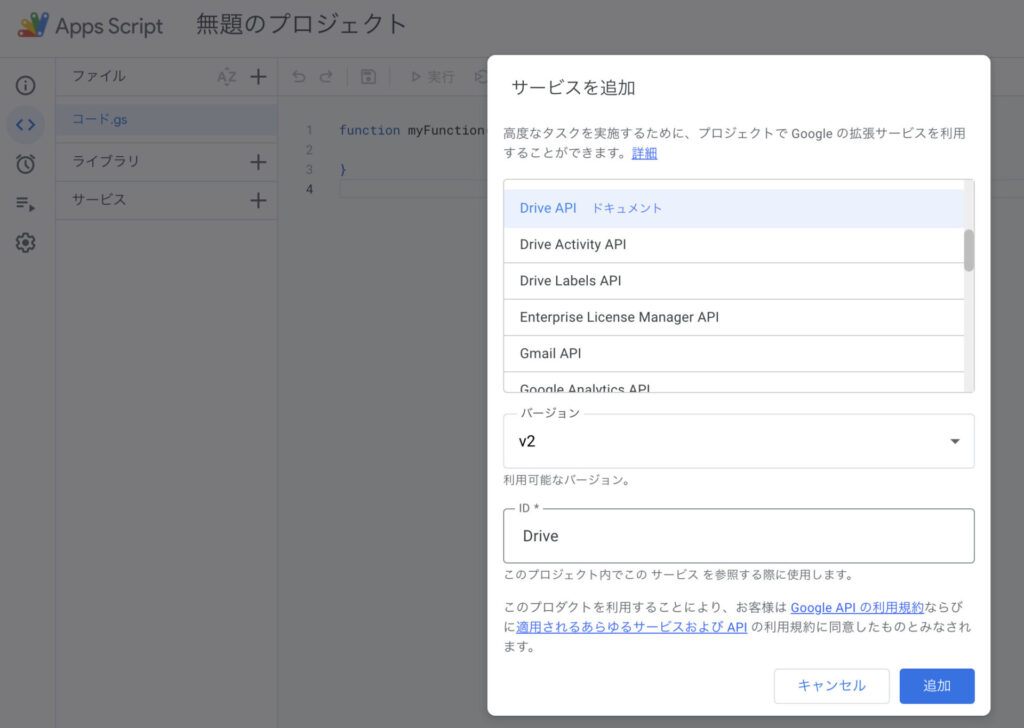
実行
あとは、コードの先頭のフォルダIDを書き換えて実行する。
実行後に、PDFが保存されているファイルをダウンロードすれば、ローカルに保存できる。
また、6分間の制限で実行が停止した場合は、コードを再実行すれば良い。